Every app in android requires certain settings for user choose and modify the way it works. The best way or the android way to handle this is using a PreferenceActivity.
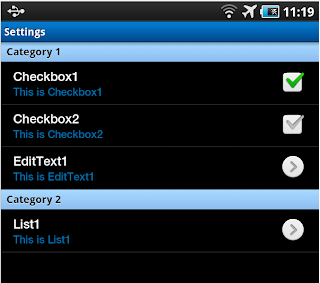
Layout Code:
For making a PreferenceActivity you first have to define a layout of it. Let’s call the layout settings.xml
/xml/settings.xml
For making a PreferenceActivity you first have to define a layout of it. Let’s call the layout settings.xml
/xml/settings.xml
<?xml version="1.0"
encoding="utf-8"?>
<PreferenceScreen xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<EditTextPreference
android:defaultValue="YOUR NAME"
android:key="namePref"
android:summary="Tell us your
name"
android:title="Name" />
<CheckBoxPreference
android:defaultValue="true"
android:key="morePref"
android:title="Enable More Settings" />
<PreferenceScreen
android:dependency="morePref"
android:key="moreScreen"
android:title="More Settings" >
<ListPreference
android:defaultValue="GRN"
android:entries="@array/color_names"
android:entryValues="@array/color_values"
android:key="colorPref"
android:summary="Choose your
favorite color"
android:title="Favorite Color" />
<PreferenceCategory android:title="Location
Settings" >
<CheckBoxPreference
android:defaultValue="true"
android:key="gpsPref"
android:summary="Use GPS to
find you"
android:title="Use GPS
Location" />
</PreferenceCategory>
</PreferenceScreen>
</PreferenceScreen>
As the ListPreference colorPref uses the array list color_names to give the user to choose from one of the colors given we need to create a string-array to give all the possible values a user may choose:
/values/arrays.xml
<?xml version="1.0"
encoding="utf-8"?>
<resources>
<string-array name="color_names">
<item>Black</item>
<item>Red</item>
<item>Yellow</item>
<item>Green</item>
</string-array>
<string-array name="color_values">
<item>BLK</item>
<item>RED</item>
<item>YEL</item>
<item>GRN</item>
</string-array>
</resources>
The layout formed above can be represented as:
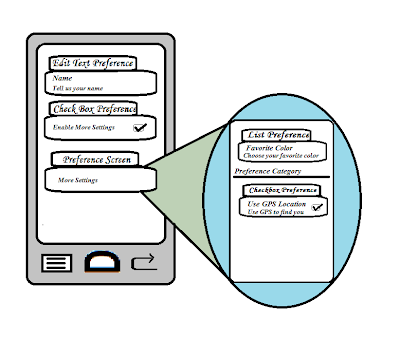
Java Code:
For Making a PreferenceActivity you will first have to create a PreferenceFragment. Which is defined as follows:
Add this activity to your AndroidManifest.xml file as follows:
/AndroidManifest.xml
For Making a PreferenceActivity you will first have to create a PreferenceFragment. Which is defined as follows:
/src/yourpackage/Prefs1Frag.java
public
class Prefs1Frag extends
PreferenceFragment
{
Context
c;
public Prefs1Frag(Context
x){c=x;}
public void onCreate(Bundle
savedInstanceState)
{
super.onCreate(savedInstanceState);
//setting default values
as in settings.xml
PreferenceManager.setDefaultValues(c, R.xml.settings, false);
addPreferencesFromResource(R.xml.settings);
}
}
Now that we have created a PreferenceFragment we need to create PreferenceActivity as follows:
/src/yourpackage/SettingsActivity.java
/src/yourpackage/SettingsActivity.java
public class SettingsActivity
extends PreferenceActivity
{
@Override
protected void onCreate(Bundle
savedInstanceState) {
super.onCreate(savedInstanceState);
getFragmentManager().beginTransaction().replace(android.R.id.content, new Prefs1Frag(this)).commit();
}
}
To get the preferences entered by the user you need this code in your Activity:
/src/yourpackage/MainActivity.java
/src/yourpackage/MainActivity.java
//getting all the preferences
SharedPreferences
settings=PreferenceManager.getDefaultSharedPreferences(this);
//getting the name preference
String name=settings.getString("namePref",
"default value");
To start the PreferenceActivity use the following code on the place which will run your preference screen:
/src/yourpackage/MainActivity.java
/src/yourpackage/MainActivity.java
public startPreferences()
{
Intent
i = new Intent(this,Prefrences.class);
this.startActivity(i);
}
Add this activity to your AndroidManifest.xml file as follows:
/AndroidManifest.xml
<activity
android:name="yourpackage.MainActivity">
</activity>
Run the code…
Run the code…