Sliding Layouts in your android app utilizes the swapping touch by the user to change the layout of your app.
/res/layout/activity_main.xml
</LinearLayout>
/src/YourPackage/MainActivity.java
/src/YourPackage/MainActivity.java
/src/YourPackage/MainActivity.java
/src/YourPackage/FirstScreen.java
Make the other two classes SecondScreen.java and ThirdScreen.java and insert layouts tab2.xml and tab3.xml respectively in the above code. Here is the picture of the projects directory structure:
Now play your project and swap the three layouts...
Layout Code
To make this project you will require 4 layout xml files (you can have more if you want more sliding layouts in your project):- activity_main.xml
- tab1.xml
- tab2.xml
- tab3.xml
activity_main.xml should contain a viewPager as shown below, all other tabs can contain any layout that you would like to display.
/res/layout/activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<android.support.v4.view.ViewPager
android:id="@+id/viewpager"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
Java Code
Your MainActivity.java class must inherit FragmentActivity instead of Activity class.
/src/YourPackage/MainActivity.java
public
class MainActivity extends
FragmentActivity
The onCreate() function of this class is defined as:
/src/YourPackage/MainActivity.java
protected void onCreate(Bundle
arg0)
{
super.onCreate(arg0);
setContentView(R.layout.activity_main);
initialisePaging();
}
The function initialisePaging() is defined as (where v is an object of ViewPager class):
/src/YourPackage/MainActivity.java
private void
initialisePaging()
{
{
List fragments = new Vector();
fragments.add(Fragment.instantiate(this, FirstScreen.class.getName()));
fragments.add(Fragment.instantiate(this, SecondScreen.class.getName()));
fragments.add(Fragment.instantiate(this, ThirdScreen.class.getName()));
v=(ViewPager)findViewById(R.id.viewpager);
PagerAdapter p=new PagerAdapter(getSupportFragmentManager(),fragments);
v.setAdapter(p);
}
Each fragment class (i.e. FirstScreen, SecondScreen, ThirdScreen for this tutorial) are defined as:
/src/YourPackage/FirstScreen.java
public class FirstScreen
extends Fragment
{
@Override
public View
onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState)
{
if(container==null)
{return null;}
return inflater.inflate(R.layout.tab1, container, false);
}
}Make the other two classes SecondScreen.java and ThirdScreen.java and insert layouts tab2.xml and tab3.xml respectively in the above code. Here is the picture of the projects directory structure:
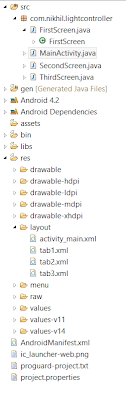
Now play your project and swap the three layouts...